A cheat sheet for Redux Toolkit with Typescript
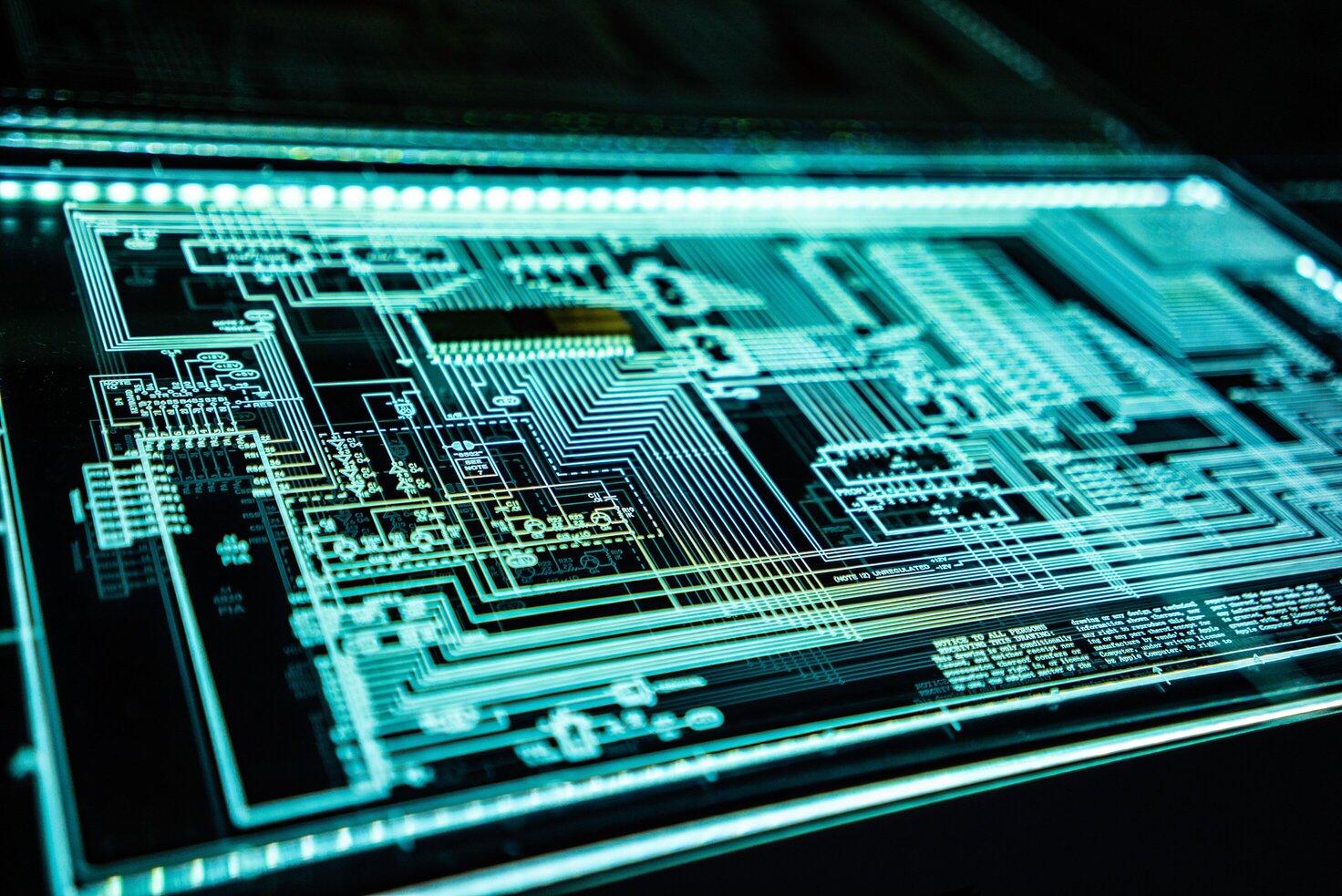
Here is a cheat sheet for Redux Toolkit with Typescript.
This is just a reference so all the bits are in one short page. The redux toolkit documentation is awesome and you should read those if this is your first time using TypeScript and Redux Toolkit together..
Note: saveState and loadState are custom methods that would put the state somewhere. like local storage.
const appReducer = combineReducers({
authentication: authSlice.reducer,
});
// Dan abramov's suggestion for clearing the state on logout
// is to create a root reducer wrapper
// note you get a type circular reference if you try to use RootState type here
const rootReducer = (state: any, action: any) => {
if (action.type === authSlice.actions.loggedOut.type) {
// Clear the state on logout if you need to
saveState({});
// and clear the state store
return appReducer(undefined, action);
}
return appReducer(state, action);
};
export const store = configureStore({
reducer: rootReducer,
// if you store the state then you can preload it here
preloadedState: loadState() as never,
});
// Infer the `RootState` and `AppDispatch` types from the store itself
export type RootState = ReturnType<typeof store.getState>;
export type AppDispatch = typeof store.dispatch;
// Create a wrapper around the useDispatch hook with the correct types
// use this in your components
export const useAppDispatch: () => AppDispatch = useDispatch;
// if you need to store the state somewhere as it changes
// https://stackoverflow.com/questions/35305661/where-to-write-to-localstorage-in-a-redux-app
store.subscribe(() => {
const state = store.getState();
saveState(state);
});
export default store;