How to add canonical meta tag in NextJs
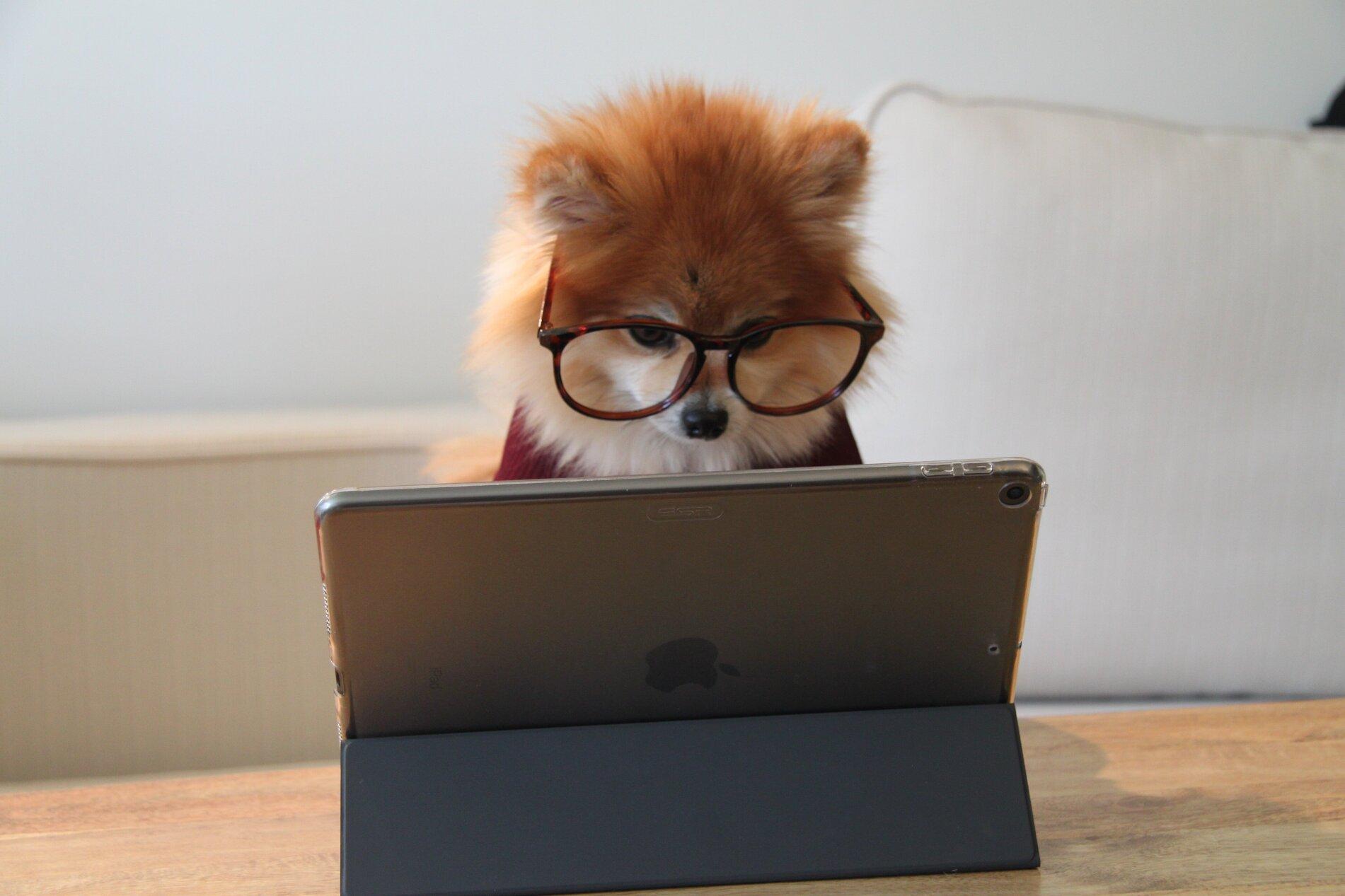
It's important to add a canonical meta tag to your pages to improve SEO or to avoid issues with query params in crawled pages.
You can easily add a canonical meta tag in NextJs by using the next/head
component.
import { useRouter } from "next/router.js";
import Head from "next/head.js";
// you need to access the route
const router = useRouter();
// then extract the path without template values (e.g. [...slug]) or query params
const canonicalUrl = (
`https://usemiller.dev` + (router.asPath === "/" ? "" : router.asPath)
).split("?")[0];
// Then somewhere in your _app.tsx or top level layout component
return (
<Head>
<link rel="canonical" href={canonicalUrl} />
</Head>
);
Alternatively, you can use the metadata export from a page or layout.
Here is use the metadataBase url. Next will prepend this to any relative urls you provide elsewhere in the meta data object.
Then set alternates.canonical
to the path you want to use as the canonical url. /
will be the base url.
export const metadata = {
metadataBase: new URL(
process.env.NEXT_PUBLIC_SITE_URL || "https://www.darraghoriordan.com"
),
alternates: {
canonical: "/",
},
};