A Nest JS Pipeline Cheatsheet
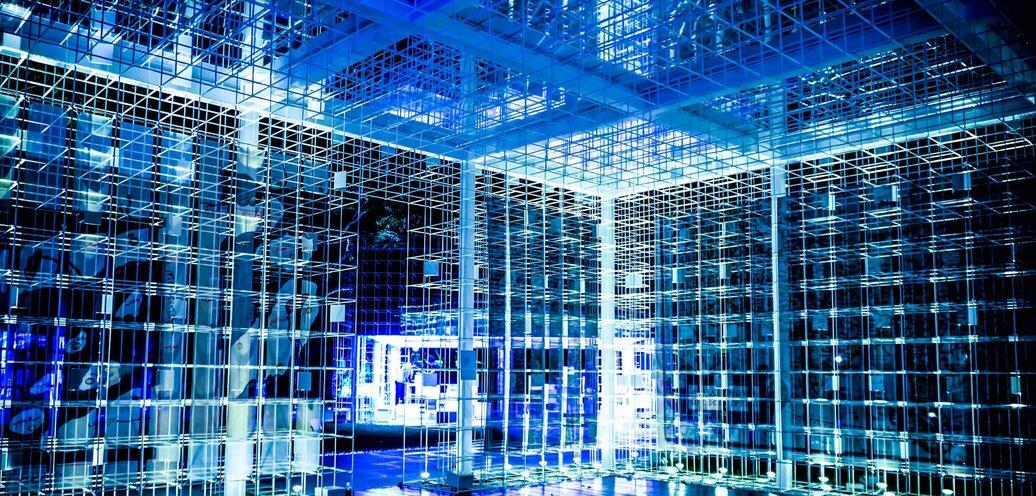
I'm always checking the NestJS documentation to read what a Middleware Vs Interceptor is and I could never remember the order that the different NestJS pipeline elements are called in.
So I made this cheatsheet for myself so I had something to refer to.
The NestJS request pipeline cheat sheet
Click to open full size image - more explanation of the cheat is available below.
In this cheat sheet I show the pipeline of Middleware -> Guards -> Before Interceptors -> Pipes -> Controllers -> Services -> After Interceptors -> Exception Filters that you would typically use in a NestJS rest application.
It's important to note that some of these are just highly focused instances of middleware or interceptors. The middleware is the most generic pipeline context and the others are just NestJS constructs to encourage convention.
This isn't 100% true because as the request goes through the pipeline more context is added and made available to you in the specific element. But I think this is a good way to think about these extra NestJS pipeline classes.
I also show where the NestJS exception zone edges are and how Decorators are related to controllers.
I add some side information about NestJS modules and application hooks.
Finally there is a list of the handy TypeORM hooks if you use TypeORM.
The Nest JS Request Pipeline
These elements are called in this order. It might be easier to see the relations in the cheat sheet diagram.
Middleware
USED FOR
Modifying or enhancing the request and response objects
Ending the request/response cycle
NOTES
Can be used to access the request and return a response.
You can’t access the result of a route.
If you return early you will bypass the rest of the NestJS pipeline
CODE
implements NestMiddleware interface
Guards
USED FOR
Used to allow or prevent access to a route
This is where you should authorise requests
NOTES
You can access the execution context here. You can check which Controller will be used
CODE
implements CanActivate interface
Before Interceptor
USED FOR
Before and after enhancing of any part of the request-response
e.g. logging or caching
NOTES
Interceptors can access the request and response
CODE
implements NestInterceptor
Pipes
USED FOR
Transforming input data
Validating input data
NOTES
Pipes should operate on the arguments to be passed to controller route.
There are a bunch of built in Pipes. ValidationPipe is very useful.
CODE
implements PipeTransform interface
Decorators
USED FOR
Extracting request data into a Controller method parameter
Or providing any data as a parameter to a controller method in a consistent way
e.g. if you set the user to the request using passport you could extract it to a custom decorator like @MyAppUser user: MyAppUser
instead of doing request?.user? every time.
NOTES
The NestJS built in decorators like @Ip()
are examples of usage
Can be composed with applyDecorators
CODE
request param decorated with @NameOfDecorator()
Controllers
USED FOR
Route handling methods
NOTES
Decorators can be applied to controllers to extract parameters from the request
CODE
decorated with @Controller()
Your Services
USED FOR
Custom business logic
NOTES
These are injected via providers.
CODE
decorated with @Injectable()
After Interceptor
USED FOR
Before and after enhancing of any part of the request-response
e.g. logging or caching
NOTES
Interceptors can access the request and response
This block represents where the interceptor handler activates BEFORE the route handler is called
CODE
implements NestInterceptor interface
Exception Filters
USED FOR
Handle thrown Errors and convert into relevant http response
NOTES
There is a built in exception filter that handles the various Nest HttpExceptions that can be thrown in your code
Exception Filters only handle exceptions thrown in the exception zone
CODE
implements ExceptionFilter interface
Additional useful NestJS hooks
Application Hooks
USED FOR
Running code in a service on application start or exit
NOTES
Turn on before app.listen() with app.enableShutdownHooks()
Use in injected service with onApplicationShutdown(signal:string)
CODE
see notes
Modules
USED FOR
Bounding and decoupling a set of domain functionality
NOTES
Has onModuleInit() and onModuleDestroy() hooks
Can be instantiated asynchronously
CODE
decorated with @Module()
Type ORM Hooks
USED FOR
Running code in a service on application start or exit
NOTES
@AfterLoad @BeforeInsert, @AfterInsert @BeforeUpdate, @AfterUpdate @BeforeRemove, @AfterRemove
CODE
only works on classes decorated with @Entity
Conclusion
Hope it helps to see these all in one place!
If you have any feedback let me know on twitter!